NAME
Game::Cribbage - Cribbage game engine
VERSION
Version 0.10
SYNOPSIS
lnation$ cribbage
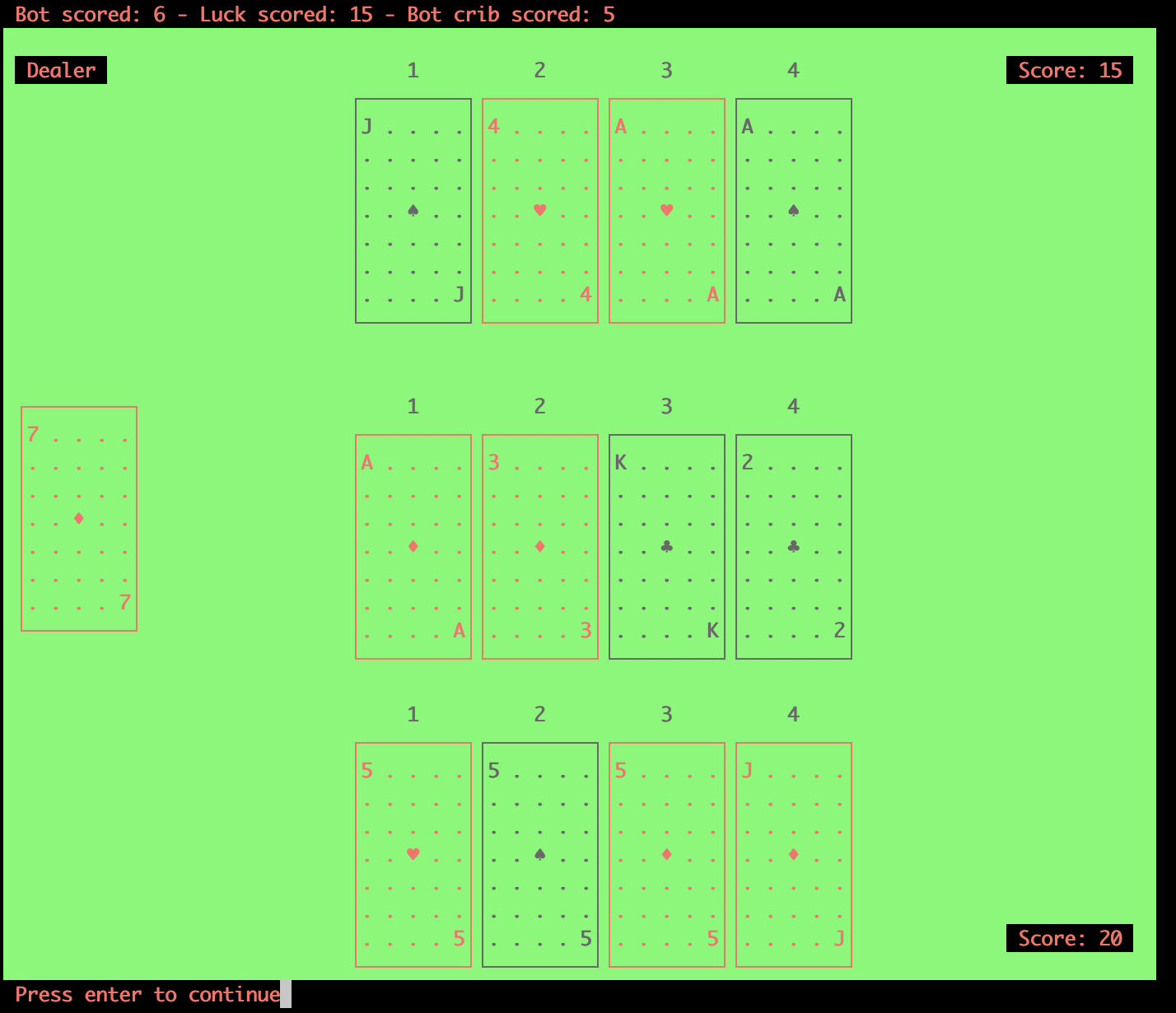
...
use Game::Cribbage::Board;
my $engine = Game::Cribbage::Board->new();
$engine->add_player(name => 'Robert');
$engine->add_player(name => 'Joseph');
$engine->start_game();
# low card logic to then set the dealer/crib player
$engine->set_crib_player('player1');
# deal hands
$engine->draw_hands();
$engine->crib_player_cards('player1', $cards);
$engine->crib_player_cards('player2', $cards);
# split to get starter
$engine->add_starter_card('player2', $card);
$engine->play_card('player1', $card);
$engine->play_card('player2', $card);
...
$engine->next_play();
...
$engine->end_hands();
$engine->score;
AUTHOR
LNATION, <email at lnation.org>
BUGS
Please report any bugs or feature requests to bug-game-cribbage at rt.cpan.org
, or through the web interface at https://rt.cpan.org/NoAuth/ReportBug.html?Queue=Game-Cribbage. I will be notified, and then you'll automatically be notified of progress on your bug as I make changes.
SUPPORT
You can find documentation for this module with the perldoc command.
perldoc Game::Cribbage
You can also look for information at:
RT: CPAN's request tracker (report bugs here)
CPAN Ratings
Search CPAN
ACKNOWLEDGEMENTS
LICENSE AND COPYRIGHT
This software is Copyright (c) 2024 by LNATION.
This is free software, licensed under:
The Artistic License 2.0 (GPL Compatible)